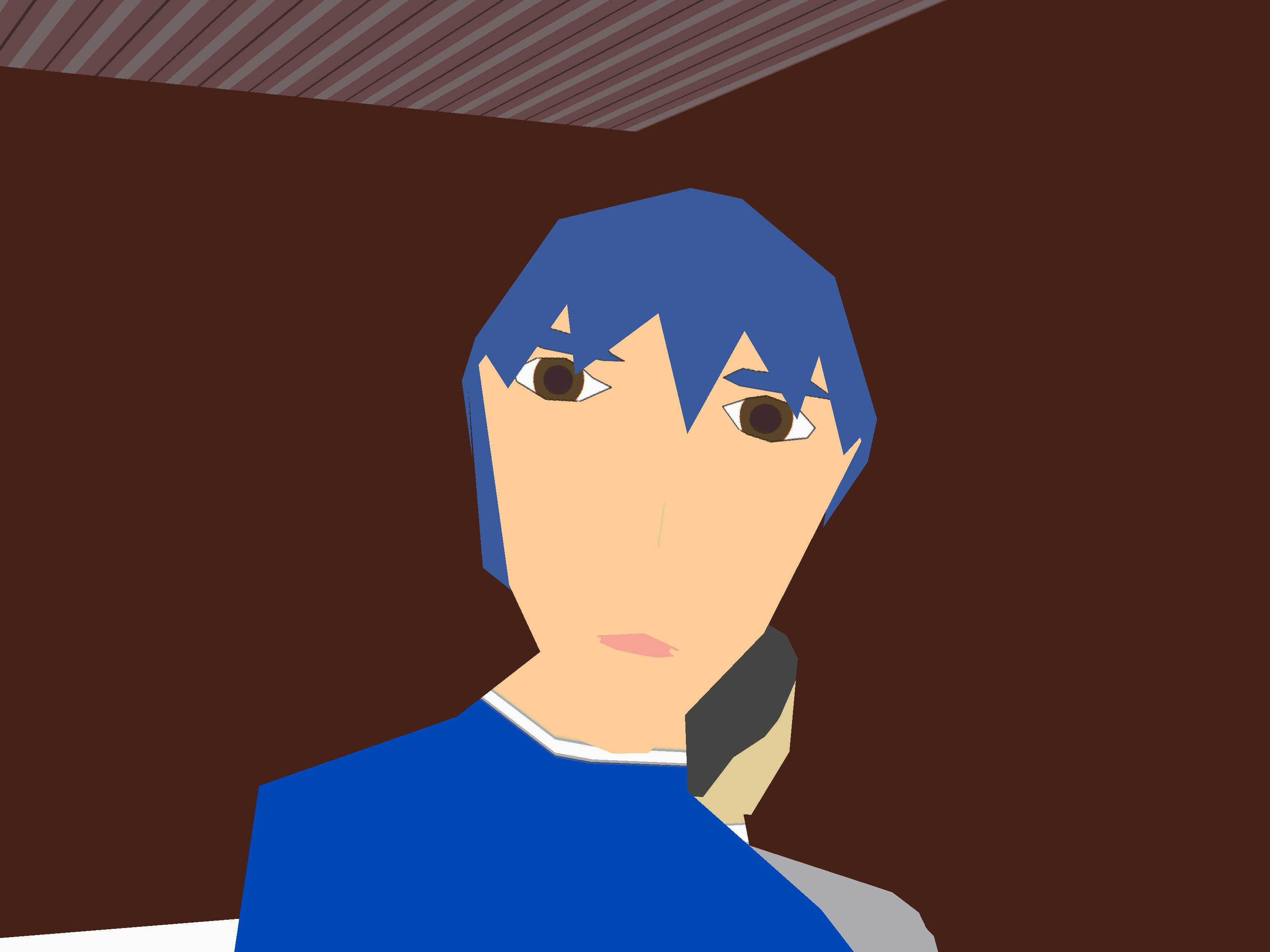
You probably didn't notice, but this is the 366th Making Javantea's Fate page. That's special because that means in 3 years I have done 1 year worth of Making Javantea's Fate pages. That's pretty amazing. It means that I've posted every third day on average. It doesn't seem possible, but it's true. I've gone months at a time without posting.
Today, I did two amazing things. First, I implemented the print resolution renderer in AltSci3D Manga Director. That means that I can render massive 3072 x 2304 images. In fact, I can have pictures of any high resolution. The code was very small and easy to add. See the code below. It's not very flexible, but flexibility can be easily built in. I'll probably use an XML attribute to set the size.
The glFrustum() is what really makes this work. Without it, I really doubt that print resolution would be possible. So the first lesson today is: there is a practical use for just about everything. Your job is to find which think is practical for what you want to do.
void AS3DFile::hiresShot() { m_eView = AS3DVIEW_PRINT_1_9; int i; for(i = 0; i < 9; i++) { render(); screenShot(); ((int)m_eView)++; } m_eView = AS3DVIEW_FIRST_PERSON; m_pCamera->setFoV(m_iWidth, m_iHeight); return; } // Change the Field of View so that it grabs only the print res tile. void AS3DCamera::setPrintRes(AS3DVIEW view) { glMatrixMode(GL_PROJECTION); // Select The Projection Matrix glLoadIdentity(); // Reset The Projection Matrix m_fTanHalfFoV = tan(AS3D_RAD*m_fFoV/2); // Calculate The Aspect Ratio Of The Window float aspect = (float)m_iWidth / (float)m_iHeight; // View Depth of 1000 float zNear = 0.1f; float zFar = 1000.0f; float nearHeight = 2.0f * zNear * m_fTanHalfFoV; float nearWidth = nearHeight * aspect; int iScale = 3; float fScale = 3; int x, y; x = (int)((view - AS3DVIEW_PRINT_1_9) / iScale); y = (int)((view - AS3DVIEW_PRINT_1_9) % iScale); float left = -nearWidth + nearWidth * x / (fScale/2); float right = -nearWidth + nearWidth * (x+1) / (fScale/2); float bottom = -nearHeight + nearHeight * y / (fScale/2); float top = -nearHeight + nearHeight * (y+1) / (fScale/2); //glFrustum(left, right, bottom, top, near, far); glFrustum(left, right, bottom, top, zNear, zFar); cout << "glFrustum(" << left << ", " << right << ", " << bottom << ", " << top << ");" << endl; glMatrixMode(GL_MODELVIEW); // Select The Modelview Matrix return; }The method I chose had just one drawback: the screenShot() outputs tiles, not a full image. The reason for not outputting the image is that it would add unnecessary complexity and memory usage (20.25 MB to be exact) to the program. I have learned that if something seems too complex and isn't necessary, it should be done by a stable program. The stable program I chose to to turn the tiles into a full image is: The GIMP 2.0. The reason I picked The GIMP is because of it's scripting language. I have tried and failed to create anything meaningful using the scripting language (even when following tutorials). So I decided to learn it again. It was much easier this time. The tutorial actually made sense. The idea of the scheme programming language is conveniently explained in this pair of haiku I made up:
Like assembly, put (instruction destination source) all are function. it lacks equal signs but a handy set! function lends itself to you.In more reasonable terms, scheme is a language of parentheses. The function name is the first argument. If there's an output, you must use the set! or let* functions and often the car function.
While this is confusing as hell, it is clear when you actually use it. Here is the first line of my script:
(set! image_all (car (gimp-image-new (* 1024 3) (* 768 3) 0)))You just do that over and over again. You can see how it's a language of parentheses, right? It gets very annoying, but modern editors have syntax highlighting, code folding, and such. It's really not that bad.
If I were writing Perl, it would look like this:
my image_all = gimp-image-new(1024 * 3, 768 * 3, 0);
So once again, I have learned a new foreign computer language in 2 days. I continue to be amazed at how similar every language is. Every language has differences and specific uses, but often coding in two different languages is a question of syntax. While the available libraries make intrinsic differences in how a person approaches each problem, library developers are commoditizing the language market. PHP has access to libraries that do compression, encryption, http, mail, graphics, databases, xml, and many other things. Python has access to even more libraries. Libraries in C++ can give access to many things that are normally exclusive to scripting languages. It's really interesting to see exactly how it is working. Developers for Microsoft VB.NET say that Longhorn has a trump card on Linux: complete dot NET implementation. Supposedly, Longhorn will be written in C#.NET, which means that it will be completely managed code. The problem with this "trump card" is that Linux already has binary compatibility with dot NET using Mono. Instead of a "trump card", this becomes the perfect assimilation of Windows programs into the GNU/Linux Operating System. If Longhorn does succeed at becoming an operating system that people can actually use, it will be just another day for Linux users using the best workstation in the world. Permalink
-
Leave a Reply
Comments: 0
Leave a reply »